C++ 文件读写常用方法
- 删除数据
- 添加数据
- 插入数据
- 替换数据
- 整数与二进制数据互转
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <io.h>
typedef unsigned char byte;
long getLength(FILE *stream) {
return filelength(fileno(stream));
}
bool removeBytes(FILE *stream, int length) {
if (length <= 0)
return true;
long pos1, pos2, oldLength, newLength, off;
pos1 = pos2 = ftell(stream);
fseek(stream, 0, 2);
oldLength = ftell(stream);
fseek(stream, pos1, 0);
newLength = oldLength - length;
off = newLength - pos1;
while (off > 0) {
int cSize = off>0x1000?0x1000:off;
char *cache = new char[cSize];
off -= cSize;
if (fseek(stream, pos2 + length, 0) ||
fread(cache, 1, cSize, stream) != cSize ||
fseek(stream, pos2, 0) ||
fwrite(cache, 1, cSize, stream) != cSize)
return false;
pos2 += cSize;
free(cache);
}
fseek(stream, pos1, 0);
return !ftruncate(fileno(stream), newLength<pos1?pos1:newLength);
}
bool addBytes(FILE *stream, int length) {
if (length <= 0)
return true;
long pos, oldLength, newLength, off;
pos = ftell(stream);
fseek(stream, 0, 2);
oldLength = ftell(stream);
fseek(stream, pos, 0);
newLength = oldLength + length;
off = oldLength - pos;
if (ftruncate(fileno(stream), newLength))
return false;
while (off > 0) {
int cSize = off>0x1000?0x1000:off;
char *cache = new char[cSize];
off -= cSize;
if (fseek(stream, pos + off, 0) ||
fread(cache, 1, cSize, stream) != cSize ||
fseek(stream, pos + length + off, 0) ||
fwrite(cache, 1, cSize, stream) != cSize)
return false;
free(cache);
}
fseek(stream, pos, 0);
return true;
}
bool insertBytes(FILE *stream, byte *bytes, long length = 4) {
if (length <= 0)
return true;
return addBytes(stream, length) && fwrite(bytes, 1, length, stream) == length;
}
bool replaceBytes(FILE *stream, long length1, byte *bytes, long length2 = 4) {
if (length1 < 0 || length2 < 0)
return true;
long length;
bool boolean;
length = length2 - length1;
if (length > 0)
boolean = addBytes(stream, length);
else if (length < 0)
boolean = removeBytes(stream, -length);
return boolean && fwrite(bytes, 1, length2, stream) == length2;
}
template<typename T>
void bytesToNumber(const uint8_t *bytes, T &n, size_t length = sizeof(T)) {
n = 0;
if (length > 0) {
for (int i = 0; i < length; ++i) {
n |= (bytes[i] & 0xff) << (length - 1 - i) * 8;
}
}
}
template<typename T>
void numberToBytes(uint8_t *bytes, T n, size_t length = sizeof(T)) {
if (bytes == nullptr) {
return;
}
if (length > 0) {
for (int i = 0; i < length; ++i) {
*bytes++ = (uint8_t)(n >> (length - 1 - i) * 8 & 0xff);
}
}
}
赏
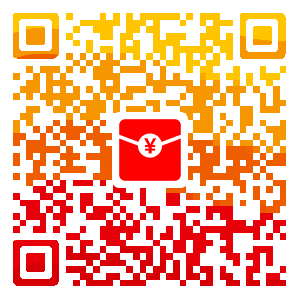
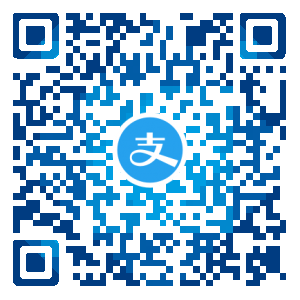
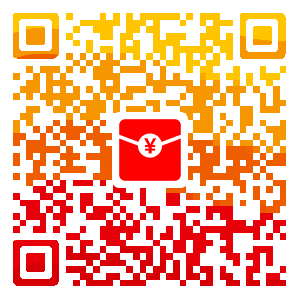
支付宝红包
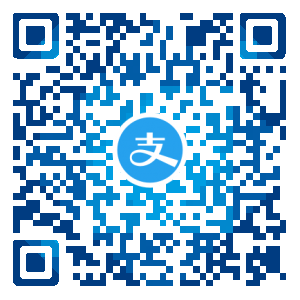
支付宝
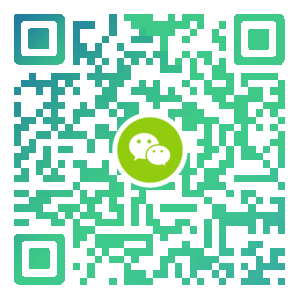
微信
可以汉化吗
回复 @小雷:
你确定没有走错场子?
回复 @小さな手は:
c#的有没有
回复 @ハッハッハ:
木有哦
没搞C#